For example, the largest international paper size of A series in photoshop is A3
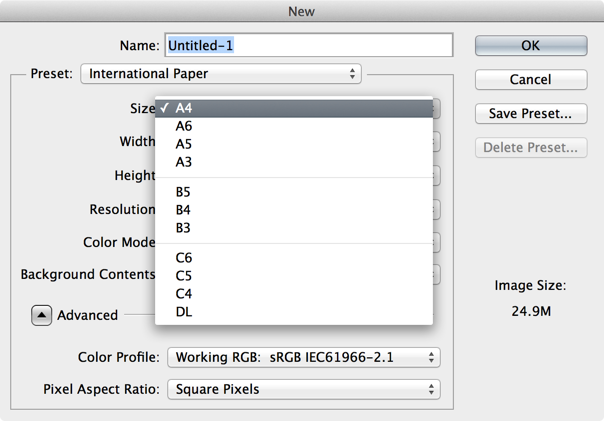
To add A2, A1, A0, we can set custom size and ‘Save Preset’.
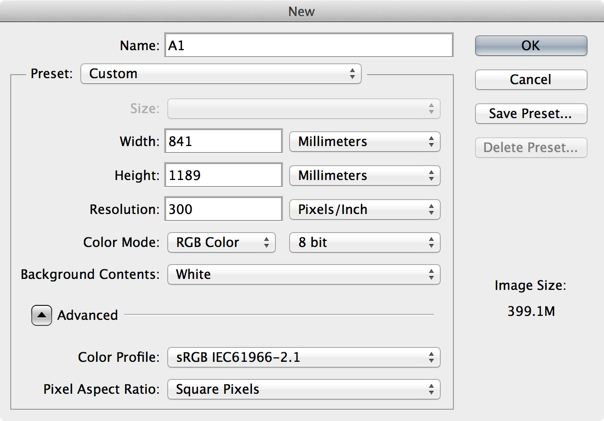
But it looks not good because photoshop don’t know they are under International Paper A Series actually.
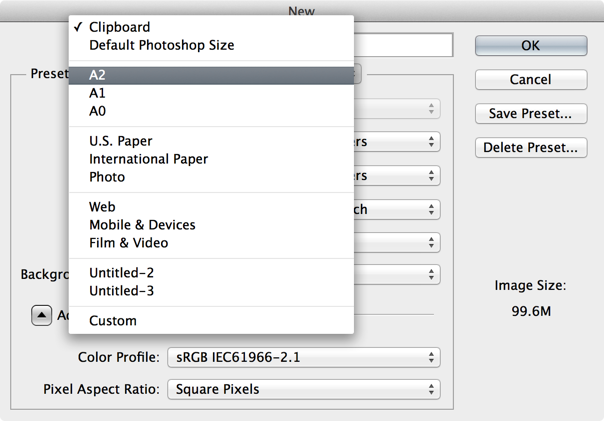
That’s better, right?
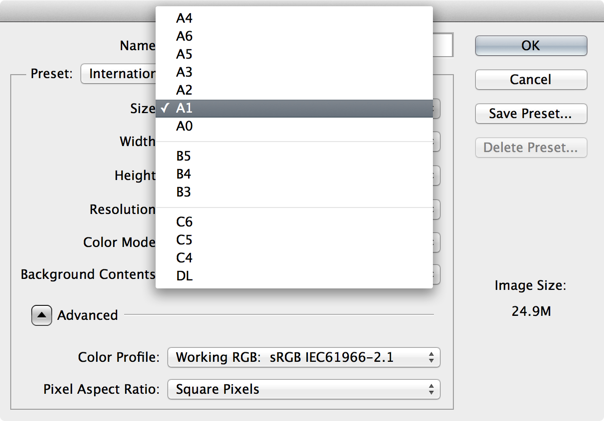
To do this, open the file ‘Default New Doc Sizes.txt’ under ‘Adobe Photoshop CC/Locales/en_US/’ and search ‘A4’, you will find:
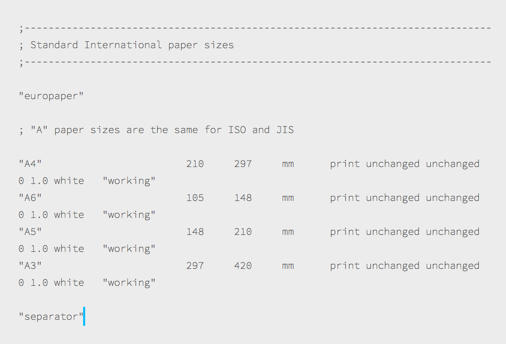
Copy, paste, edit
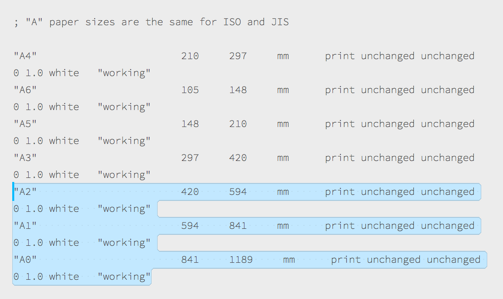
Quit & relaunch photoshop, that’s it, you can add more missing size now.
p.s. the location of ‘Default New Doc Sizes.txt’ depends on what version and lauguage of Photoshop we’ve installed